Saturday morning update, hoping to get a few hours in across today and tomorrow.
Not much change on the pinball (interesting bit), but plenty of things to help get the code going. The iterations were taking too long for me: , deploying to the device and then taking it to the machine, powering up, testing, monitoring the console line, turn off, back to code etc, etc -> therefor I have just completed the following:
I have implemented over the air (OTA) updates using a web browser. Lots of guides on t'internet to do this, but its really simple with the ESP32 to do this. (
https://randomnerdtutorials.com/esp32-over-the-air-ota-programming/)
As I now have a web server running on the ESP32, I may as well write a single web page application (SPA) that can be used to read info on the machine states and trigger a few switches virtually.
Its not as complex as you would think, I create an empty web page, based on the brilliant W3CSS templates (
https://www.w3schools.com/w3css/w3css_templates.asp). Then using simple AJAX calls (basically JavaScript in the page opening web urls, reading the response and then updating elements in the web page, real time) to get variables from the software, or to initiate functions in the code.
A quick chat about the method for this:
- In a separate header file, import the web libraries and start the server.
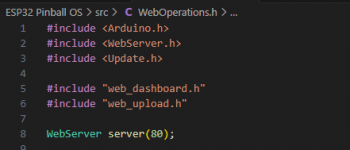
The web_dashboard.h and web_upload.h are the files with the HTML of my web pages.
- Define the function for the web operations, I'll be using this as a task pinned to a core later

- Define the functions that will do the work when web urls are accessed.
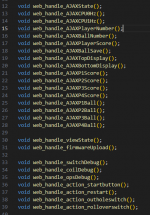
- Setup the task handler we will use for the task we pin to the core.

- The WebOperationsFunction - these bits set up the listeners that can respond to URL calls. The bits in quotes is the URL, the bits without brackets are the action to take if the URL is hit. So basically, I'm calling functions. This is like the setup function in the main Arduino file, we will only call it once.
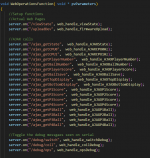
- A few more of these listener setups and then the code that handles the firmware upload.
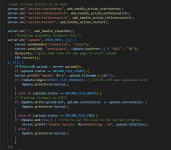
- Then we make sure we dont spend our afternoon waiting for Wi-Fi to connect
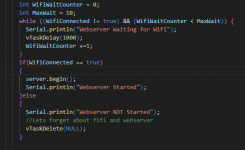
- The vTaskDelete(NULL) basically destroys the web server elements if we cant get Wi-Fi connected. Why have it running if its not usable.
- Then the looped bit of the task. The vTaskDelay(2) is important as without it, the ESP32 crashes because the task consumes too much compute time the onboard processes cant run. The delay allows them time to run.
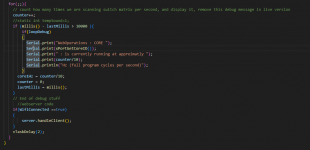
- We then go back to the main program file and setup the task in the setup() function
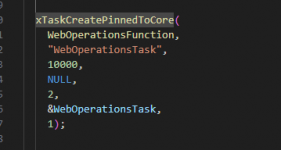
- Finally the actual functions called in the listeners need their programming.
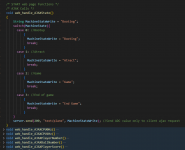
As you can see, its really primitive at the moment, its not going to help responsiveness when the game is actually in use, but will be invaluable to help me focus on developing the important bits of the code and staying still.
Looking forward to making some progress this weekend, its been a few weeks since I have had capacity to get on with it
BUGS TO FIX:
ESP32 boards seem to have a flaw where when running Wi-Fi and only using the 5v in through board pins, it can brown out really easily. I have seen this. The second you only power via USB or add USB power its all good. Done some research and I think I'll need to connect the ground of the USB port to another ground pin to solve. I'll give this a go later down the line as I'm not going to be using it without a USB connected laptop for a while.
EDIT: Other progress
Started using GitHub to hold my code and manage the to-do list that gets longer and longer
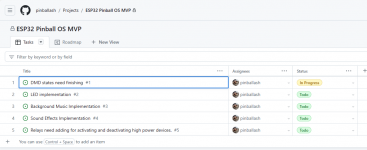